Whenever I think I invent something - it means I haven’t google enough.
Let continue digging to a trivial whiteboard task.
So top 1 quote on the interview about the trivial task.
I use …. library for it.
Reminder
Array uniqueFrom last time our winner is a method that uses maps for search. We get O(N) complexity.
1 | function uExtraMap (arr) { |
Why Reinvent a wheel?
Libraries are written and maintained by the big community. It is written by mega smart folks much smarter than you.
Just use XXXX lib
In general, it is true. It is faster to use well tested and checked the library.
The dark side of the lib
- It is designed to be a lib. Wide generic cases covered
- It is intended to serve library cases and compositions. It follows patterns of lib.
- The size.
- hidden complexity
Lets take 2 top Folks
It is merely one-liners in every library. Simple and elegant.
Lodash
https://lodash.com/ is a seasoned and old continuation of underscore.
1 | _.uniq(a10000) |
What inside ?!
source code
So as we see 80 lines with banch of imports.
As we could guess from names - sophisticated set implementation is used.
Ramda
Ramda more functional pure and super popular
More fun why
1 | R.uniq(a10000) |
What inside ?!
As we could see the code is even quite close to what we have))
uniqBy
1 | var set = new _Set(); |
Let’s take a look to graph.
Ups ((
The Price
So let’s remove all IE7 unfriendly cases from our test and all deviations of map implementation.
We want to compare or winner with a lodash and ramda.
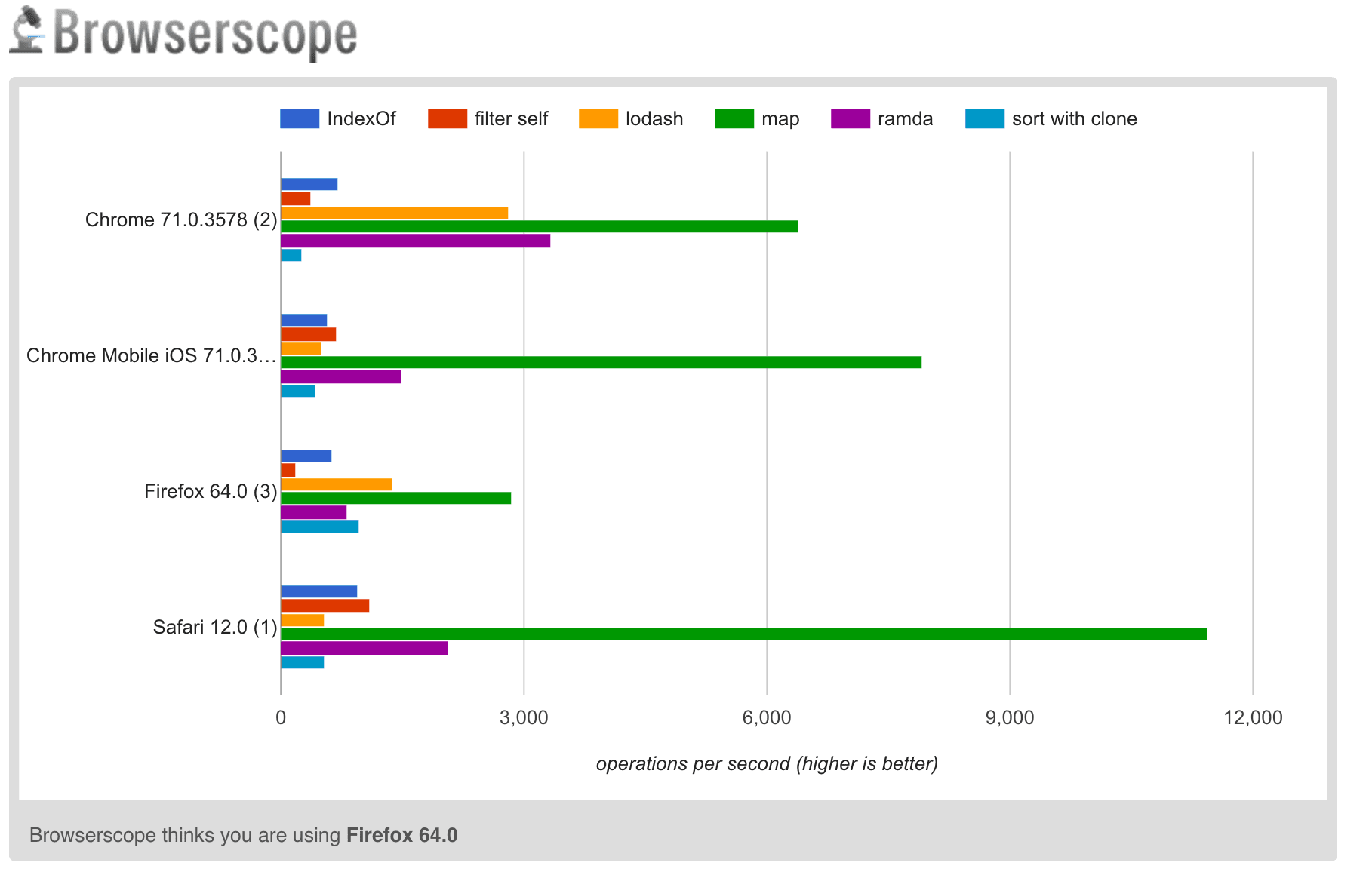
jsperf test give all code and allow to test on your machine.
As we can see Ramda do much better but still far away.
It is dost mean that our solution is super smart and better, rather opposite. We can see that for concrete cases less mean more. I believe that lodash or ramda code is quite a cold evening reading with a lot of closed cases and sophisticated cool logic and corner cases.
The key question here - is it part of our problem?
Libraries are a focus on general cases.
The lesson learn
- Read the code of your libraries
- Understand your code
- Understand key KPI and requirement of your system or even “Trivial task.”
- Do not trust the library gods
- The dark side of dark side - if you spend 80% time to tune your little helper … maybe you are writing yet another monster.
PS
One more cool reading about a topic.
You-Dont-Need-Lodash
As I said, It is not black and white. I showed why ES6 Implementation is not always cool.
Wait wait I use babel
You don’t need to struggle that Old browsers do not support cool stuff
1 | function es6Unique (arr) { |
Lets use babel
but let’s have a chat next time about it…